basic in c programming
in the first place, C is a general-purpose programming language, which was designed by Dennis Ritchie in 1972 and C is not a very high-level language
in reality, It will be little difficult to understand C if we compare with a high-level language like python
on this occasion, suppose, if you are planning to learn C++, C#, and Java it is better to learn C first
But, why?
because C++, C#, and Java are based on C.
Why C is called a system programming language?
Because it is used for writing compilers and operating systems
In other words, C language is used for writing operating systems due to its closeness to the hardware
in brief, C is a small language
But, why do you say it is a small language?
Because C offers only single thread control flow tests, subprograms(recursion,function), loops, statement grouping
on the other hand, C does not support multiprogramming, parallel operations, coroutines, and synchronization
There are only 32 keywords available in C but when we compare them to C++ keywords, every new standard[C++11, C++14, C++17, C++20] has new keywords
on the whole, C is not a strongly-typed language
But, why do you say C is a weekly typed language?
To illustrate that C is a weekly typed language I will use two concepts
- Data type conversion
- Function prototypes
Data type conversion
For example: In weekly typed programming language if we subtract an integer with string at that instant, the code will get executed but with some warning even though it is a data type mismatch
But the same thing is not possible in strongly typed programming languages like C++ where the compiler will through an error
But,why?
Because of the data type mismatch
The strongly typed language will throw an error: explicit (outside) conversion is required while on the other hand, the weekly-typed language will compile the code by implicit (inside) that is, data type conversion done by the compiler
to demonstrate, a C example that is, for the subtraction of an integer with a string
#include <stdio.h>
int sub_two(int x, int y){ //Function
return x - y;
}
int main(){
int a=12, sub;//integer
char b[]="sub";//string
sub = sub_two(a, b); //function call
// Displaying output
printf("subtraction of two items: %d", sub);
return 0;
}
Output
subtraction of two items:-2686712
this time, a C++ example for the subtraction of an integer with a string
#include <iostream>
using namespace std;
int sub_two(int x, int y){ //Function
return x - y;
}
int main()
{
int a=12, sub;//integer
char b[]="sub";//string
//function call
sub = sub_two(a, b); // error: invalid conversion from char* to 'int'
// Displaying output
cout << "subtraction of two items:" <<sub<<endl;
return 0;
}
Function prototypes
as a matter of fact, in C you can call the function pow() without including the header file #include<math.h>
but with little warning
Whereas in C++ you can not call a function pow() without including the header file #include<math.h>
But, why?
Because C++ has strong type checking that doesn’t allow any function call without its function prototype #included
#include <stdio.h>
//#include <math.h>
int main()
{
int a=2,b=3,c;
c=pow(a,b);//warning implicit declaration of function 'pow'
printf("power=%d",c);
return 0;
}
Output
power=8
at this time, a C++ example to show that if we did not include a file called #include<math.h> in the program then, in that case, if we called the function pow() will result in an error
#include <iostream>
//#include <math.h>
using namespace std;
int main()
{
int a=2,b=3;//error 'pow' was not declared in this scope if you did not file #include<math.h>
cout << "power=" <<pow(a,b)<< endl;
return 0;
}
The drawback of C language
in essence, the major problem with C language is its concept called pointer
on one hand, Pointer errors are difficult to find, and pointers are not secure from the security and safety point of view
on the other hand, Pointers are the main culprits for memory leakage in the program
in truth, Java is created to avoid the notorious pointers bugs of C/C++
Difference between low-level language C and high-level language Python
C program
#include <stdio.h>
int main()
{
printf("Hello world!\n");
return 0;
}
Python program
print('Hello, world!')
at this point, well, imagine this
for instance, suppose, you like to print hello world! With low-level language C and high-level language Python
Next, when you wrote a C program to print hello world! you need 6 lines of instruction and while, on the other hand, you just need one line of instruction to print hello world! With Python
in this situation, can you deconstruct the difference between the low-level language C and the high-level language Python?
in fact, Python is pretty effective, right?
But, why?
Because you need just one line of instruction in python to express yourself to complete the task
On the other hand, in C you need 6 lines of instructions to express yourself to complete the required task
So, what’s the conclusion?
High-level languages like Python are effortlessly easy to write and read as well as shorter
If that is true, then why do we need C which is a nightmare to read and write as well as long
Because C is a compiled language that means C will execute the instructions faster when compared with Python
so far, clear as mud?
Difference between C and C++
C program
#include <stdio.h>
int main()
{
printf("Hello world!\n");
return 0;
}
C++ program
#include <iostream>
using namespace std;
int main()
{
cout << "Hello world!" << endl;
return 0;
}
Here’s another one
at this instant, suppose, you like to print hello world! With C and C++
until now, okay,
in short, Yes! We wrote hello world! Programm in C and C++
with this in mind, next, what?
to sum up, can you spot the difference between C and C++ hello world! program
of course, No!
But, Why?
Because they look quite similar to each other right?
Yes! Because both C and C++ are low-level languages and any valid C program is also a C++ program
Then what is the difference?
C++ means it is an extension of C with some added features such as OOPS
Writing a program in C
- Define the program objectives
- Design the program
- Write code
- Compile
- Run the program
- Test and debug
- Maintain the program
Standard header files
Structure of a C program
Main( )
{
Statement 1;
.......
.....n;
}
Function 1( )
{
Statement 1;
.........
.......n;
}
Function 2( )
{
Statement1;
.......
....n;
}
Function N( )
{
Statement 1;
..........
.......n;
}
Writing a simple C program
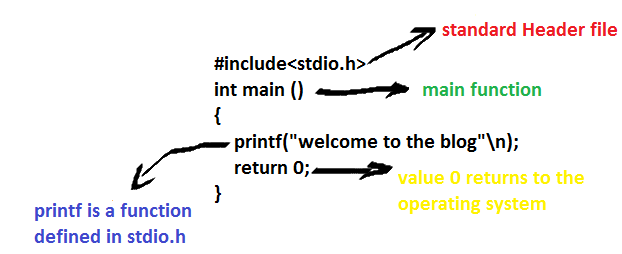
on one hand, Return 0 means the integer value return by main() function indicates success in compiling the given code
on the other hand, Return a non zero if the main() function fail to do its intended work. next, that indicates failure in compiling the given code
Statement terminator
that is to say, every statement you write with c program must be terminated with a semicolon(;)
Input and output function
not to mention, scanf () function receives input values and printf() function print output values on the screen
Syntax of a printf() function
Printf("<format string>",<no of variables>);
<format string> contain
Int uses %d
Float uses %f
Char uses %c
Character string uses %s
Example
Printf("%d", x);
scanf("%f%f%d",&x,&y,&z);
We use scanf() to receive input values from user
Example
Scanf("%d",&x);
printf("%f%f%d", x,y,z);
Note: the difference between printf() and scanf() function
use address operator(&) in front of variable for scanf() function
What is a main() in c program?
It is a function, which you can consider as a vessel with a set of statements in it.
Int main()
{
Statement1;
...................
..................n;
}
The datatype int kept in front of main() function to return an integer number
How to comment in a c program?
Comments in a c program must be enclosed within asterisk(*)
/* this is a comment*/
resources
- Let us C by Yashavant Kanetkar
- Let us C++ by Yashavant Kanetkar
- The C programming language by Brian w.Kernighan Dennis M.Ritchie
- Python programming an introduction to computer science by John Zelle
Leave a Reply