Let’s build a project that will help us, wirelessly to read the temperature in Celsius, Fahrenheit and also humidity, heat index by using ESP32 Board and DHT11 sensor
The project creates a webserver that will update reading automatically without refreshing the webpage so, that you can see the live reading with the current time, date, month, year
Have you ever fermented any food in your life? If you don’t then let me share with you how it is done?
Fermented food such as sauerkraut, cucumber pickles, jalapeno pickles contain probiotics live bacteria that provide powerful gut health and ease the digestive system to digest the nutrition from the vegetables
During the fermentation process, temperature plays a very important role, if the temperature is high then it will speed up the fermentation process, plus also simultaneously, reduces the fermentation days and the fermented food contains live probiotics bacteria.
If the temperature exceeds 30 degrees Celsius, During the fermentation process, it can kill the healthy probiotic bacteria and can damage the vegetable
So, because of that, keeping an eye on temperature helps us, a lot during the fermentation process, especially for people living in hot climate countries such as India where the temperature in summer months can shoot up to 44 degrees Celcius
Usually, it is recommended to use glass jars for fermentation because they are more nonreactive than steel and plastic but when it comes to hot climate countries earthen jars play an important role
Why?
because the earthen jar can reduce the outer temperature and above that by using our esp32 web server will let you know the exact temperature of outer environment and inner environment temperature of earthen jars
with the help our esp32 webserver you will know when to use the earthen jar and glass jar to protect the vegetable from getting damage
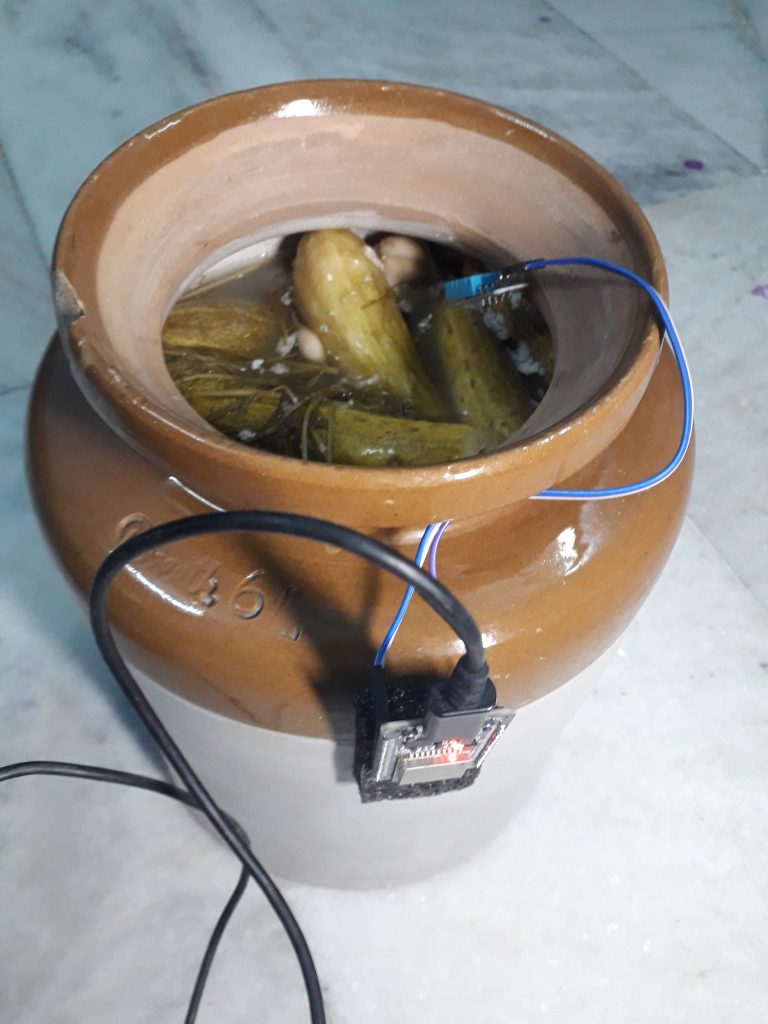
How to use the code?
You need to install a couple of libraries for this project
Once you downloaded the listed libraries in .ziped format then at that instant make sure you unzipped it
Next, rename the folders to
DHT-Sensor-library- master to DHT_Sensor
Adafruit_Sensor-master to Adafruit_Sensor
ESPAsyncWebServer-master to ESPAsyncWebServer
AsyncTCP-master to AsyncTCP
Finally, move the renamed libraries folders to the Arduino IDE installation libraries folder
Schematic
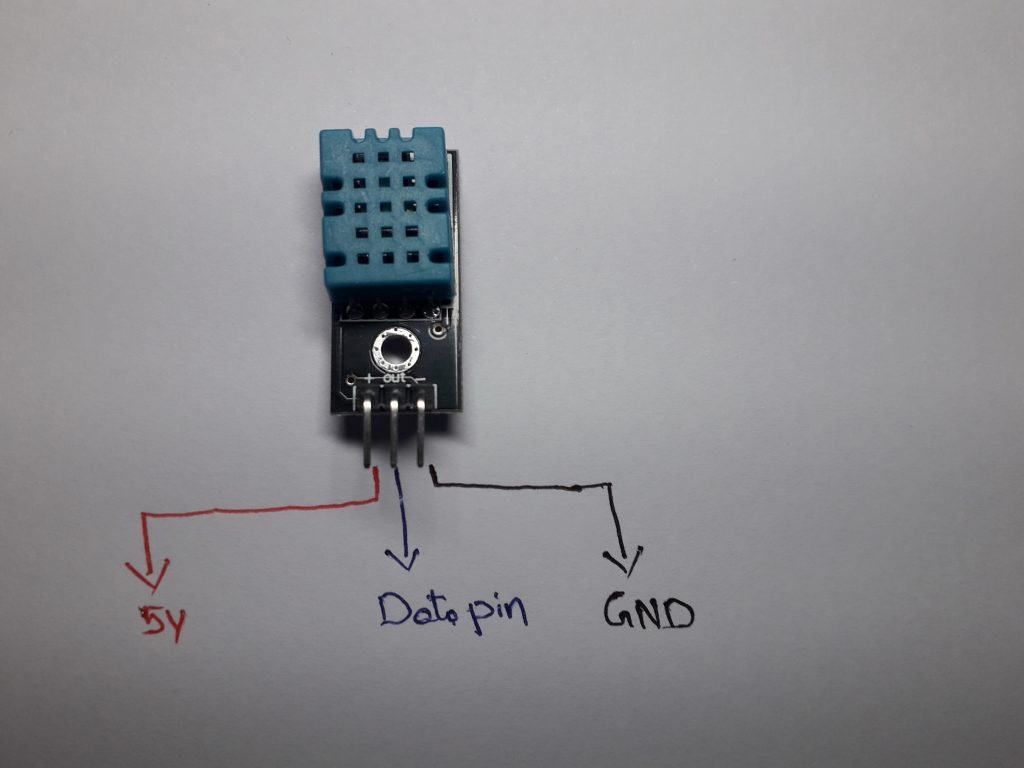
Take three female to female jumper wires
Connect DHT11 sensor (-) pin to the theESP32 Board GND pin
Connect DHT11 sensor (out) pin to the ESP32 Board pin 27
As the ESP32 board has a 3v3 volt pin so, connect the DHT11 sensor (+) pin to the 3v3 pin of the ESP32 Board
If all the libraries are set properly then the next step is to compile the program in your Arduino ide and when the code is uploaded successfully on the board then at that instant, open the serial monitor to view the IP address
In case, if you see a blank screen when you open the serial monitor then at that instant, you need to press the EN button from the ESP32 board
At last, type the IP address in the web browser of your device so, that you can see the live reading of the DHT11 sensor
// Import required libraries
#include "WiFi.h"
#include "ESPAsyncWebServer.h"
#include <Adafruit_Sensor.h>
#include <DHT.h>
// Replace with your network credentials
const char* ssid = "xx";
const char* password = "%ssid6630&";
#define DHTPIN 27 // Digital pin connected to the DHT sensor
// Uncomment the type of sensor in use:
#define DHTTYPE DHT11 // DHT 11
//#define DHTTYPE DHT22 // DHT 22 (AM2302)
//#define DHTTYPE DHT21 // DHT 21 (AM2301)
DHT dht(DHTPIN, DHTTYPE);
// Create AsyncWebServer object on port 80
AsyncWebServer server(80);
String readDHTTemperature() {
// Sensor readings may also be up to 2 seconds 'old' (its a very slow sensor)
// Read temperature as Celsius (the default)
float t = dht.readTemperature();
// Read temperature as Fahrenheit (isFahrenheit = true)
//float t = dht.readTemperature(true);
// Check if any reads failed and exit early (to try again).
if (isnan(t)) {
Serial.println("Failed to read from DHT sensor!");
return "--";
}
else {
Serial.println(t);
return String(t);
}
}
String readDHTHumidity() {
// Sensor readings may also be up to 2 seconds 'old' (its a very slow sensor)
float h = dht.readHumidity();
if (isnan(h)) {
Serial.println("Failed to read from DHT sensor!");
return "--";
}
else {
Serial.println(h);
return String(h);
}
}
String readDHTFahrenheit() {
// Sensor readings may also be up to 2 seconds 'old' (its a very slow sensor)
float f = dht.readTemperature(true);
if (isnan(f)) {
Serial.println("Failed to read from DHT sensor!");
return "--";
}
else {
Serial.println(f);
return String(f);
}
}
String computeDHTHeatIndex() {
// Sensor readings may also be up to 2 seconds 'old' (its a very slow sensor)
float t = dht.readTemperature();
float h = dht.readHumidity();
float hic = dht.computeHeatIndex(t, h, false);
{
Serial.println(hic);
return String(hic);
}
}
String readDHTComment() {
// Sensor readings may also be up to 2 seconds 'old' (its a very slow sensor)
// Read temperature as Celsius (the default)
float T = dht.readTemperature();
// Read temperature as Fahrenheit (isFahrenheit = true)
//float t = dht.readTemperature(true);
{
if(T <= 12.00) {
return "Too cold to ferment!";
}
// else if((T >=18.00)&&(T<=21.00)) {
// return "Ideal for ferment ";
// }
else{
if(T>=29.00) {
return "Too hot to ferment ! ";
}
else{
return "Ideal for ferment ";
}
}
}
}
const char index_html[] PROGMEM = R"rawliteral(
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width, initial-scale=1">
<style>
body {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0px auto;
font-family: 'Open Sans', sans-serif;
background: #222;
background-image: url('https://source.unsplash.com/1600x900/?landscape');
font-size: 180%;
}
.card {
background:#000000d0 ;
color: white;
padding: 2em;
width: 100%;
max-width: 200px;
border-radius: 30px;
}
.flex {
display: flex;
align-items: center;
}
.description {
text-transform: capitalize;
margin-left: 8px;
}
<!--clock start here-->
.datetime{
background: #000000d0;
color: white;
padding: 15px 10px;
font-family: "Segoe UI", sans-serif;
width:340px;
border: 3px solid #2E94E3;
border-radius: 5px;
}
.date{
font-size: 18px;
font-weight: 600;
text-align: center;
letter-spacing: 1px;
color: #fff;
background:#000000d0 ;
padding-top: 20px;
}
.time{
font-size: 40px;
display: flex;
justify-content:center ;
align-items:center ;
color: #fff;
background: #000000d0;
padding-top: 10px;
}
.time span:not(:last-child){
position: relative;
margin: 0 6px;
font-weight: 600;
text-align: center;
letter-spacing: 3px;
}
.time span:last-child{
background: #2E94E3;
font-size: 30px;
font-weight: 600;
text-transform: uppercase;
margin-top: 10px;
padding: 0 5px;
border-radius: 3px;
}
</style>
<script type="text/javascript">
function updateClock(){
var now = new Date();
var dname = now.getDay(),
mo = now.getMonth(),
dnum = now.getDate(),
yr = now.getFullYear(),
hou = now.getHours(),
min = now.getMinutes(),
sec = now.getSeconds(),
pe = "AM";
if(hou >= 12){
pe = "PM";
}
if(hou == 0){
hou = 12;
}
if(hou > 12){
hou = hou - 12;
}
Number.prototype.pad = function(digits){
for(var n = this.toString(); n.length < digits; n = 0 + n);
return n;
}
var months = ["January", "February", "March", "April", "May", "June", "July", "Augest", "September", "October", "November", "December"];
var week = ["Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday"];
var ids = ["dayname", "month", "daynum", "year", "hour", "minutes", "seconds", "period"];
var values = [week[dname], months[mo], dnum.pad(2), yr, hou.pad(2), min.pad(2), sec.pad(2), pe];
for(var i = 0; i < ids.length; i++)
document.getElementById(ids[i]).firstChild.nodeValue = values[i];
}
function initClock(){
updateClock();
window.setInterval("updateClock()", 1);
}
</script>
<script>
setInterval(function ( ) {
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
document.getElementById("temperature").innerHTML = this.responseText;
}
};
xhttp.open("GET", "/temperature", true);
xhttp.send();
}, 10000 ) ;
setInterval(function ( ) {
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
document.getElementById("humidity").innerHTML = this.responseText;
}
};
xhttp.open("GET", "/humidity", true);
xhttp.send();
}, 10000 ) ;
setInterval(function ( ) {
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
document.getElementById("fahrenheit").innerHTML = this.responseText;
}
};
xhttp.open("GET", "/fahrenheit", true);
xhttp.send();
}, 10000 ) ;
setInterval(function ( ) {
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
document.getElementById("heat_index").innerHTML = this.responseText;
}
};
xhttp.open("GET", "/heat_index", true);
xhttp.send();
}, 10000 ) ;
setInterval(function ( ) {
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
document.getElementById("comment").innerHTML = this.responseText;
}
};
xhttp.open("GET", "/comment", true);
xhttp.send();
}, 10000 ) ;
</script>
</head>
<body onload="initClock()">
<!--digital clock start-->
<div class="time"></div>
<div class="datetime">
<div class="date">
<span id="dayname">Day</span>,
<span id="month">Month</span>
<span id="daynum">00</span>,
<span id="year">Year</span>
</div>
<div class="time">
<span id="hour">00</span>:
<span id="minutes">00</span>:
<span id="seconds">00</span>
<span id="period">AM</span>
</div>
<div class="card" style="background:#000000d0;color: white; padding: 2em;">
<h2 >Temp in <sup class="units">°C</sup> and<sup class="units">°F</sup></h2>
<div class="flex"> <span id="temperature" style="font-size: 24px;">%TEMPERATURE%</span><sup class="units"style="font-size: 24px;">°C   </sup> <span style="font-size: 24px;">| </span><span id="fahrenheit" style="font-size: 24px;">%FAHRENHEIT%</span><sup class="units" style="font-size: 24px;">°F  </sup></div>
<div class="flex" >
<img src="https://openweathermap.org/img/wn/04n.png" alt="" class="icon" />
<div> <span id="comment">%COMMENT%</span></div>
</div>
<div><span class="dht-labels">Humidity: </span><span id="humidity">%HUMIDITY%</span><sup class="units">%</sup></div>
<div><span class="dht-labels">Heat index: </span><span id="heat_index">%HEATINDEX%</span> <sup class="units">°C</sup></div>
</div>
</div>
</body>
</html>)rawliteral";
// Replaces placeholder with DHT values
String processor(const String& var){
//Serial.println(var);
if(var == "TEMPERATURE"){
return readDHTTemperature();
}
else if(var == "HUMIDITY"){
return readDHTHumidity();
}
else if(var == "FAHRENHEIT"){
return readDHTFahrenheit();
}
else if(var == "HEATINDEX"){
return computeDHTHeatIndex();
}
else if(var == "COMMENT"){
return readDHTComment();
}
return String();
}
void setup(){
// initialize serial:
Serial.begin(115200);
Serial.print("Connecting to ");
Serial.println(ssid);
dht.begin();
// Connect to Wi-Fi
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.print(".");
}
// Print local IP address of your network
Serial.println("");
Serial.println("WiFi connected.");
Serial.println("IP address: ");
Serial.println(WiFi.localIP());
// Route for root / web page
server.on("/", HTTP_GET, [](AsyncWebServerRequest *request){
request->send_P(200, "text/html", index_html, processor);
});
server.on("/temperature", HTTP_GET, [](AsyncWebServerRequest *request){
request->send_P(200, "text/plain", readDHTTemperature().c_str());
});
server.on("/humidity", HTTP_GET, [](AsyncWebServerRequest *request){
request->send_P(200, "text/plain", readDHTHumidity().c_str());
});
server.on("/fahrenheit", HTTP_GET, [](AsyncWebServerRequest *request){
request->send_P(200, "text/plain", readDHTFahrenheit().c_str());
});
server.on("/heat_index", HTTP_GET, [](AsyncWebServerRequest *request){
request->send_P(200, "text/plain", computeDHTHeatIndex().c_str());
});
server.on("/comment", HTTP_GET, [](AsyncWebServerRequest *request){
request->send_P(200, "text/plain", readDHTComment().c_str());
});
// Start server
server.begin();
}
void loop(){
}
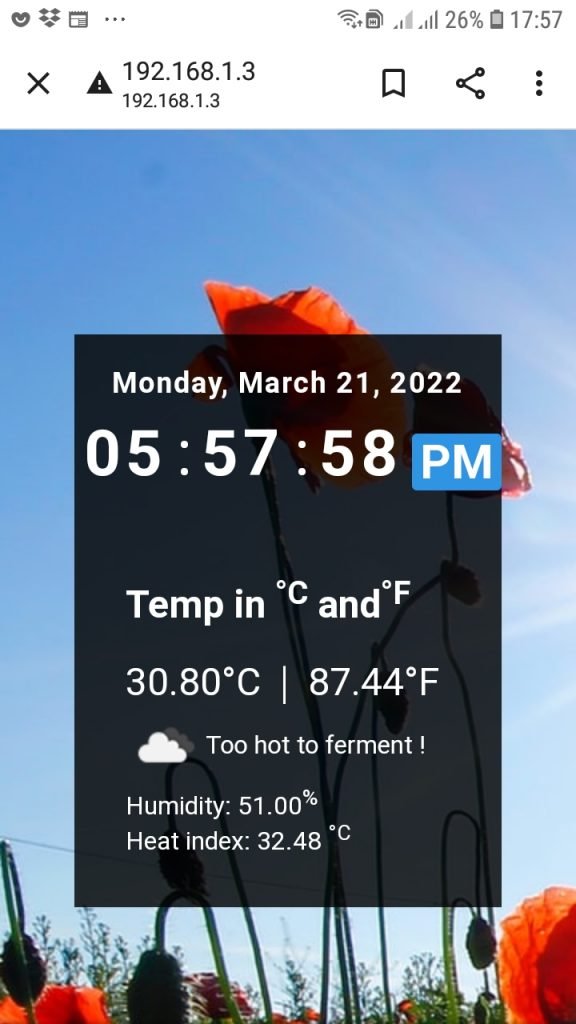
Leave a Reply